As I’ve been delving deeper into JavaScript, I’ve found myself starting local projects, wanting to get into the habit of making my commits and pushing them to GitHub. So, you’ve created a folder for your little project; now how do you get it onto GitHub?
Table of Contents
Initializing the Local Repo in Git
Once you’ve created your project’s folder, you’ll then want to open Terminal and CD into the folder. Now you’ll initialize the repo in Git with the following command:
git init -b main
At this this time, you can then create a file new file called .gitignore
in TextEdit on a macOS, or nano/vim/vi
in Terminal. This file allows you to specify various files, folders, or filetypes to ignore when adding files and making commits. Here is an example of what you can put in you .gitignore
file:
# Ignore Mac system files
.DS_store
# Ignore all zip files
*.zip
# Ignore tmp folder
/tmp
Now you can add all files in the local project folder to git
with the following command:
git add .
The first commit can bow be made by running:
git commit -m “The first commit”
Creating the GitHub Repository
We’re not ready to create the repository on GitHub that will be used to push the local commits to.
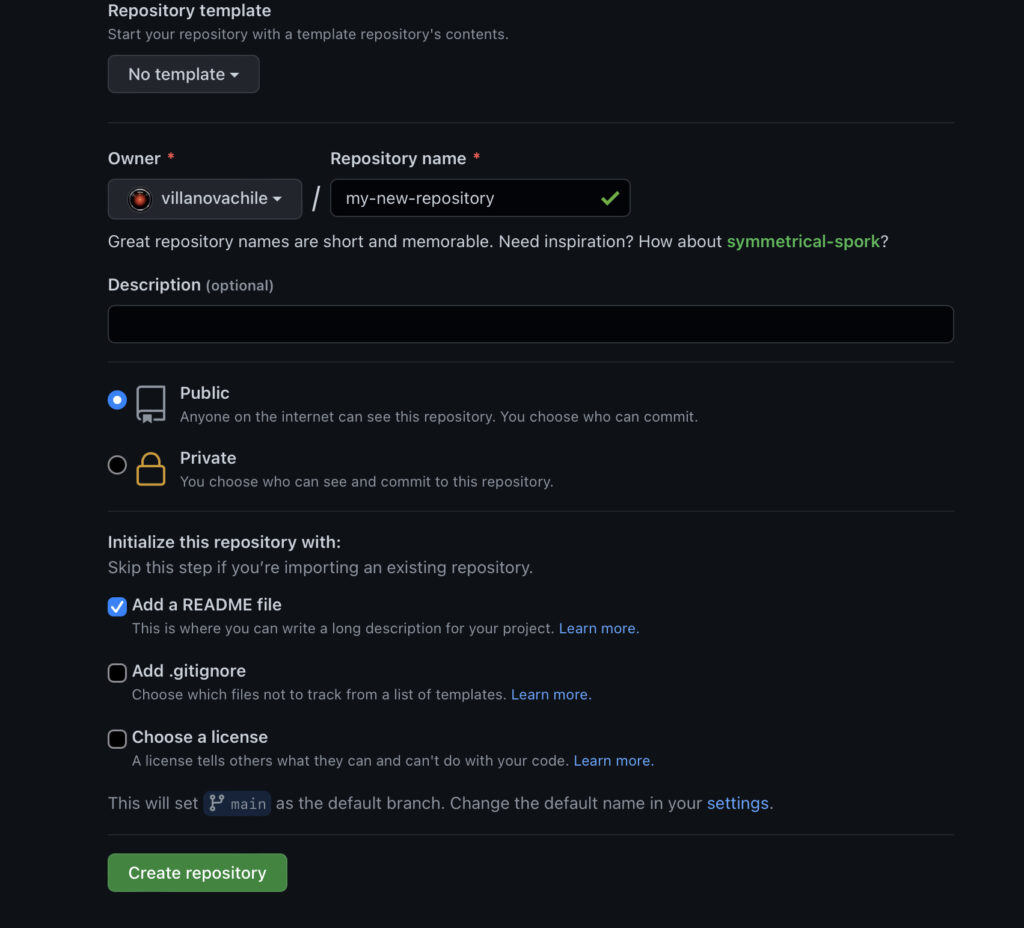
Once that has been created, you’ll then want to get the SSH link to your repository. This can be done by navigating to your newly created repository on GitHub, then clicking the green Code button, choosing the SSH option, and clicking the copy button.
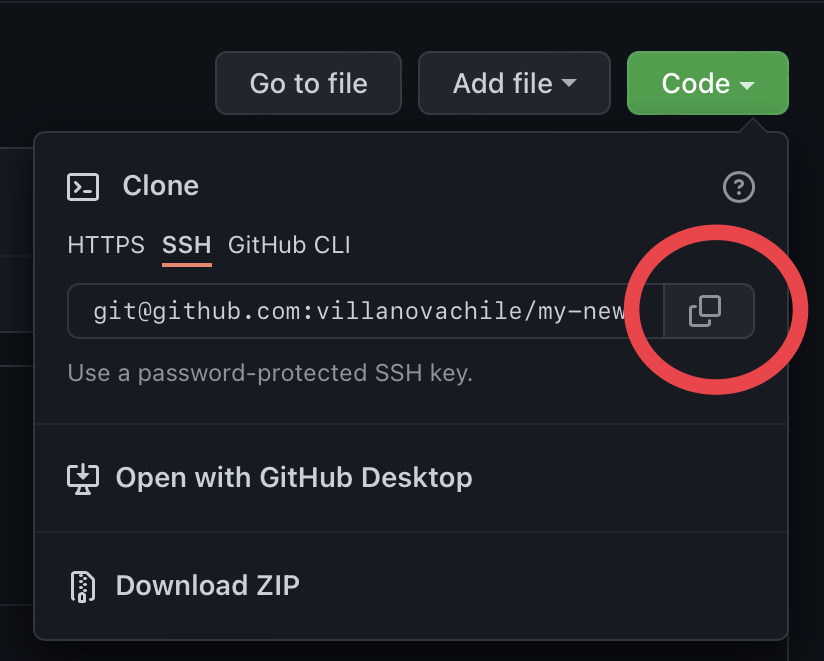
The HTTPS
link will work just fine, however, it will prompt you for your login/password every time you make changes. If you have have your SSH public key set up as described in this article, then you’ll want to use the SSH link.
Pushing the Project to GitHub
The next step will be to add the URL for the remote repository, which is where you want the files to be pushed to on GitHub. This can be done by running, and pasting the SSH link we copied from above.
git remote add origin <SSHLink>
You can verify the URL by then entering:
git remote -v
The final step will be to push the local files to your GitHub repository by running:
git push -u origin main
If you’ve selected the option to create a README when creating your repository on GitHub, then you may see this message:
! [rejected] main -> main (fetch first)
error: failed to push some refs to 'github.com:villanovachile/my-new-repository.git'
hint: Updates were rejected because the remote contains work that you do
hint: not have locally. This is usually caused by another repository pushing
hint: to the same ref. You may want to first integrate the remote changes
hint: (e.g., 'git pull ...') before pushing again.
hint: See the 'Note about fast-forwards' in 'git push --help' for details.
This essentially means that since there is a file in the remote repository that is not present in the local repository, you must first make a pull by running:
git pull origin main
If you see the error fatal: refusing to merge unrelated histories then you can can add the --allow-unrelated-histories
tag such as:
git pull origin main --allow-unrelated-histories
You should now see any existing files from GitHub in your local repo folder, and you can run the following push command again:
git push -u origin main
You should now be able to refresh your GitHub repository and see your local files!